Push Notification
Android
If you haven't already done the setup in Firebase and Kommunicate dashboard, follow the steps below:
For firebase setup and to get you server key follow this section.
Now go to Kommunicate dashboard Push Notification section, add the Server key obtained from firebase dashboard under GCM/FCM key and save.
Download the google-services.json
file from the firebase dashboard and paste it under <YourApp>/android/app/
directory.
Download KmFirebaseMessagingService.java file and place it under <YourApp>/android/app/src/main/java/io/pushnotification/
directory.
Add KmFirebaseMessagingService.java
entry inside the <application
tag in <YourApp>/android/app/src/main/AndroidManifest.xml
file as below (Refer here):
<service
android:name="io.pushnotification.KmFirebaseMessagingService"
android:exported="true"
android:stopWithTask="false" />
Finally, follow these steps:
Goto <YourApp>/android/build.gradle
- Add the following under buildscript -> dependencies
classpath 'com.google.gms:google-services:3.0.0'
- Add the following at the bottom of the file:
apply plugin: 'com.google.gms.google-services'
After adding, it will look something like this:
buildscript {
repositories {
mavenCentral()
jcenter()
}
...
dependencies {
classpath 'com.android.tools.build:gradle:2.2.1'
classpath 'com.google.gms:google-services:3.1.1'
}
}
...
apply plugin: 'com.google.gms.google-services'
iOS
Upload your APNS certificates for both development and distribution under Kommunicate dashboard Push Notification/iOS section. And update capabilities in Xcode as described here.
Swift
Open the project in XCode
Create new file with name
KommunicateWrapper.swift
inios/Runner/
.Copy the code for
KommunicateWrapper.swift
from this link and paste it inside your newly created file.Open
<YourApp>/ios/Runner/AppDelegate.swift
file, if the file does not exists, create new one. Add the below code in the file:
import UIKit
import Flutter
import UserNotifications
@UIApplicationMain
@objc class AppDelegate: FlutterAppDelegate {
override func application(
_ application: UIApplication,
didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?
) -> Bool {
GeneratedPluginRegistrant.register(with: self)
UNUserNotificationCenter.current().delegate = self
KommunicateWrapper.shared.application(application, didFinishLaunchingWithOptions: launchOptions)
return super.application(application, didFinishLaunchingWithOptions: launchOptions)
}
override func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
KommunicateWrapper.shared.userNotificationCenter(center, willPresent: notification, withCompletionHandler: { options in
completionHandler(options)
})
}
override func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
KommunicateWrapper.shared.userNotificationCenter(center, didReceive: response, withCompletionHandler: {
completionHandler()
})
}
override func applicationWillTerminate(_ application: UIApplication) {
KommunicateWrapper.shared.applicationWillTerminate(application: application)
}
override func applicationWillEnterForeground(_ application: UIApplication) {
print("APP_ENTER_IN_FOREGROUND")
UIApplication.shared.applicationIconBadgeNumber = 0
}
override func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
print("DEVICE_TOKEN_DATA :: \(deviceToken.description)") // (SWIFT = 3) : TOKEN PARSING
KommunicateWrapper.shared.application(application, didRegisterForRemoteNotificationsWithDeviceToken: deviceToken)
}
}
Objective-C
Open your Flutter project.
Run
flutter pub get
.Open the iOS file of your project in Xcode.
Create a new file named
KommunicateWrapper.Swift
in the "Runner" folder.Xcode may prompt you to create a bridging header file. If so, click on "Create."
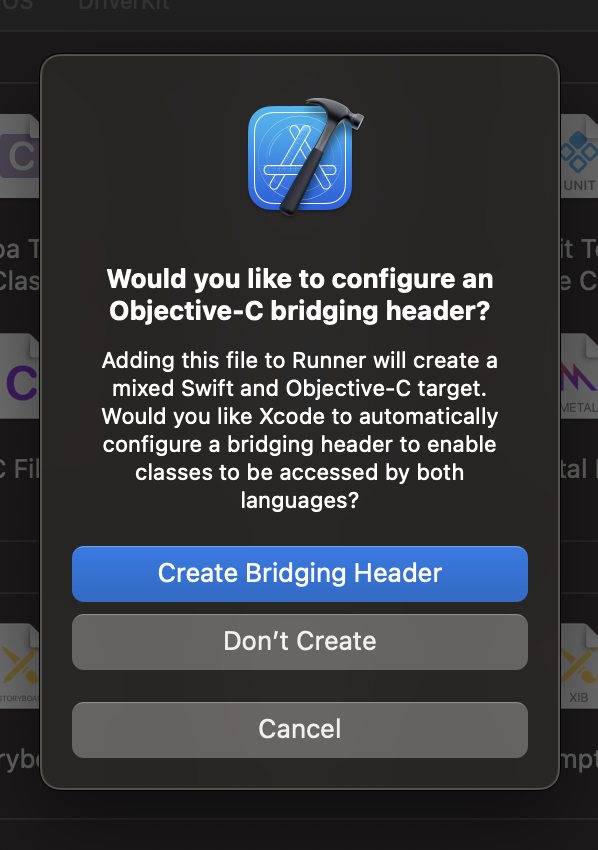
Add the code from this Link to your
KommunicateWrapper.Swift
file.Go to your AppDelegate.m file import Runner-Swift.h & the Bridging Header File.
Add the below exposed notification code to your AppDelegate.m file.
#import "AppDelegate.h"
#import "Kommunicate-Swift.h"
#import "KommunicateObjcSample-Swift.h"
#import <UserNotifications/UserNotifications.h>
@interface AppDelegate () <UNUserNotificationCenterDelegate>
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
[UNUserNotificationCenter.currentNotificationCenter setDelegate:self];
return [KommunicateWrapper.shared application:application didFinishLaunchingWithOptions:launchOptions];
}
- (void)applicationWillResignActive:(UIApplication *)application {
// Sent when the application is about to move from active to inactive state. This can occur for certain types of temporary interruptions (such as an incoming phone call or SMS message) or when the user quits the application and it begins the transition to the background state.
// Use this method to pause ongoing tasks, disable timers, and invalidate graphics rendering callbacks. Games should use this method to pause the game.
}
- (void)applicationDidEnterBackground:(UIApplication *)application {
[KommunicateWrapper.shared applicationDidEnterBackground:application];
}
- (void)applicationWillEnterForeground:(UIApplication *)application {
[KommunicateWrapper.shared applicationWillEnterForeground:application];
}
- (void)applicationDidBecomeActive:(UIApplication *)application {
// Restart any tasks that were paused (or not yet started) while the application was inactive. If the application was previously in the background, optionally refresh the user interface.
}
- (void)applicationWillTerminate:(UIApplication *)application {
[KommunicateWrapper.shared applicationWillTerminateWithApplication:application];
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken
{
[KommunicateWrapper.shared application:application didRegisterForRemoteNotificationsWithDeviceToken:deviceToken];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions))completionHandler {
[KommunicateWrapper.shared userNotificationCenter:center willPresent:notification withCompletionHandler:^(UNNotificationPresentationOptions options) {
completionHandler(options);
}];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[KommunicateWrapper.shared userNotificationCenter:center didReceive:response withCompletionHandler:^{
completionHandler();
}];
}
@end
Note: If you are using Kommunicate in an Objective-C app, then check this repo sample app in Objective-C. Create a wrapper file in Swift and call the functions in the wrapper from Objective-C files in your Project.
Using flutter firebase messaging plugin
If you are using firebase_messaing plugin in your app for push notifications, follow the below steps to make Kommunicate notifications work with the Firebase plugin:
Open
KmPushNotificationService.java
and extends it withFlutterFirebaseMessagingService
instead ofFirebaseMessagingService
In the
KmPushNotificationService.java
file add the below import:
import io.flutter.plugins.firebase.messaging.FlutterFirebaseMessagingService;
- In AndroidManifest.xml file add or replace the below service inside <application tag:
<service
android:name="io.pushnotification.KmFirebaseMessagingService"
tools:node="replace"
android:exported="true"
android:stopWithTask="false">
<intent-filter>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
Add the below tools entry (If not already added) inside the <manifest tag of AndroidManifest.xml file as below:
xmlns:tools="http://schemas.android.com/tools"
for example (2nd line in the below snippet):
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
package="io.kommunicate.kommunicate_flutter_plugin_example">
- Add the below entry in within the <application tag in your AndroidManifest.xml file:
<service
android:name="io.flutter.plugins.firebasemessaging.FlutterFirebaseMessagingService"
tools:node="remove"/>